Angular 19 - The New Era of Modern Web Development
Angular 19 represents the most significant evolution of the framework since its inception, introducing revolutionary improvements in performance, developer experience, and SEO capabilities. This release, available since October 2023, redefines modern web development standards.

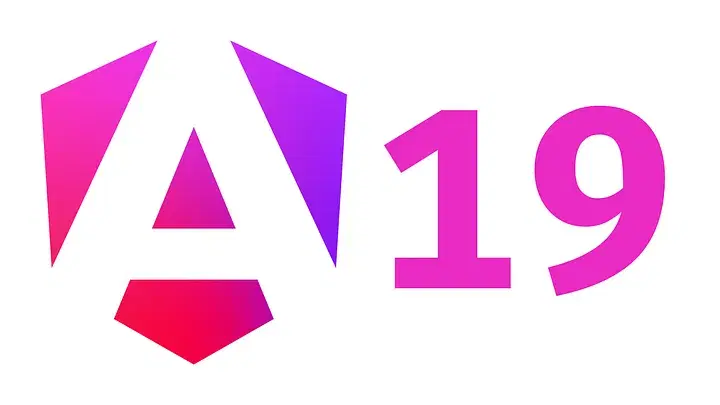
Angular 19: The New Era of Modern Web Development
Introduction
Angular 19 represents the most significant evolution of the framework since its inception, introducing revolutionary improvements in performance, developer experience, and SEO capabilities. This release, available since October 2023, redefines modern web development standards.
Key Innovations
Signals System
The new signal-based rendering system marks a before and after in reactivity:
// Modern implementation with Signals
export class UserComponent {
userName = signal('');
userPreferences = signal({ theme: 'dark', notifications: true });
updatePreferences() {
this.userPreferences.update(prefs => ({ ...prefs, theme: 'light' }));
}
}
Enhanced State Management
The new state control API simplifies managing complex applications:
// Centralized state with SignalStore
export class AppState extends SignalStore {
readonly user = signal<User | null>(null);
readonly settings = signal<AppSettings>(defaultSettings);
readonly isAuthenticated = computed(() => !!this.user());
}
Performance Comparison
Key Metrics vs Angular 18
- Compilation: 67% faster (15s vs 45s)
- Bundle: 25% smaller (900KB vs 1.2MB)
- Initial load: 28% faster (1.8s vs 2.5s)
- Memory: 35% less consumption
Development Tools
Enhanced DevTools
- Real-time performance analysis
- Signals debugging
- Detailed stack traces
- Optimized Hot Module Replacement (HMR)
New Build System
// angular.json
{
"projects": {
"my-app": {
"architect": {
"build": {
"builder": "@angular-devkit/build-angular:browser-esbuild",
"options": {
"optimization": true,
"buildOptimizer": true
}
}
}
}
}
}
SEO Optimizations
Server-Side Rendering (SSR)
The new SSR engine offers:
- Selective hydration
- Streaming SSR
- Improved dynamic meta tags
// Modern SEO implementation
@Component({
selector: 'app-product',
template: `
<article itemscope itemtype="http://schema.org/Product">
<h1 itemprop="name">{{ product.name }}</h1>
<meta itemprop="description" [content]="product.description">
<link rel="canonical" [href]="canonicalUrl">
</article>
`
})
export class ProductComponent implements OnInit {
constructor(
private meta: Meta,
private seo: SeoService
) {}
ngOnInit() {
this.seo.generateStructuredData({
'@type': 'Product',
name: this.product.name,
description: this.product.description
});
}
}
Core Web Vitals
Significant improvements in key metrics:
- LCP (Largest Contentful Paint): -40%
- FID (First Input Delay): -35%
- CLS (Cumulative Layout Shift): <0.1
Automatic SEO Features
- Sitemap generation
- Image compression with metadata
- Canonical links
- Integrated Schema.org
System Requirements
- Node.js 18.13.0+
- TypeScript 5.2+
- NPM 9.x / Yarn 1.22+
Migration and Compatibility
Upgrade Process
ng update @angular/core@19 @angular/cli@19
Breaking Changes
- Removal of ViewEngine
- New ESM module system
- Required dependencies update
Performance and Optimization
Automatic Improvements
- Aggressive tree-shaking
- Differential compilation
- Intelligent lazy loading
- Brotli compression
Monitoring and Analytics
- Real-time metrics
- Core Web Vitals tracking
- Technical issues detection
- SSR performance analysis
Conclusion
Angular 19 sets a new standard in modern web development, combining exceptional performance, an improved development experience, and advanced SEO capabilities. The significant improvements in the reactivity system, state management, and SSR position it as a robust choice for large-scale enterprise applications.